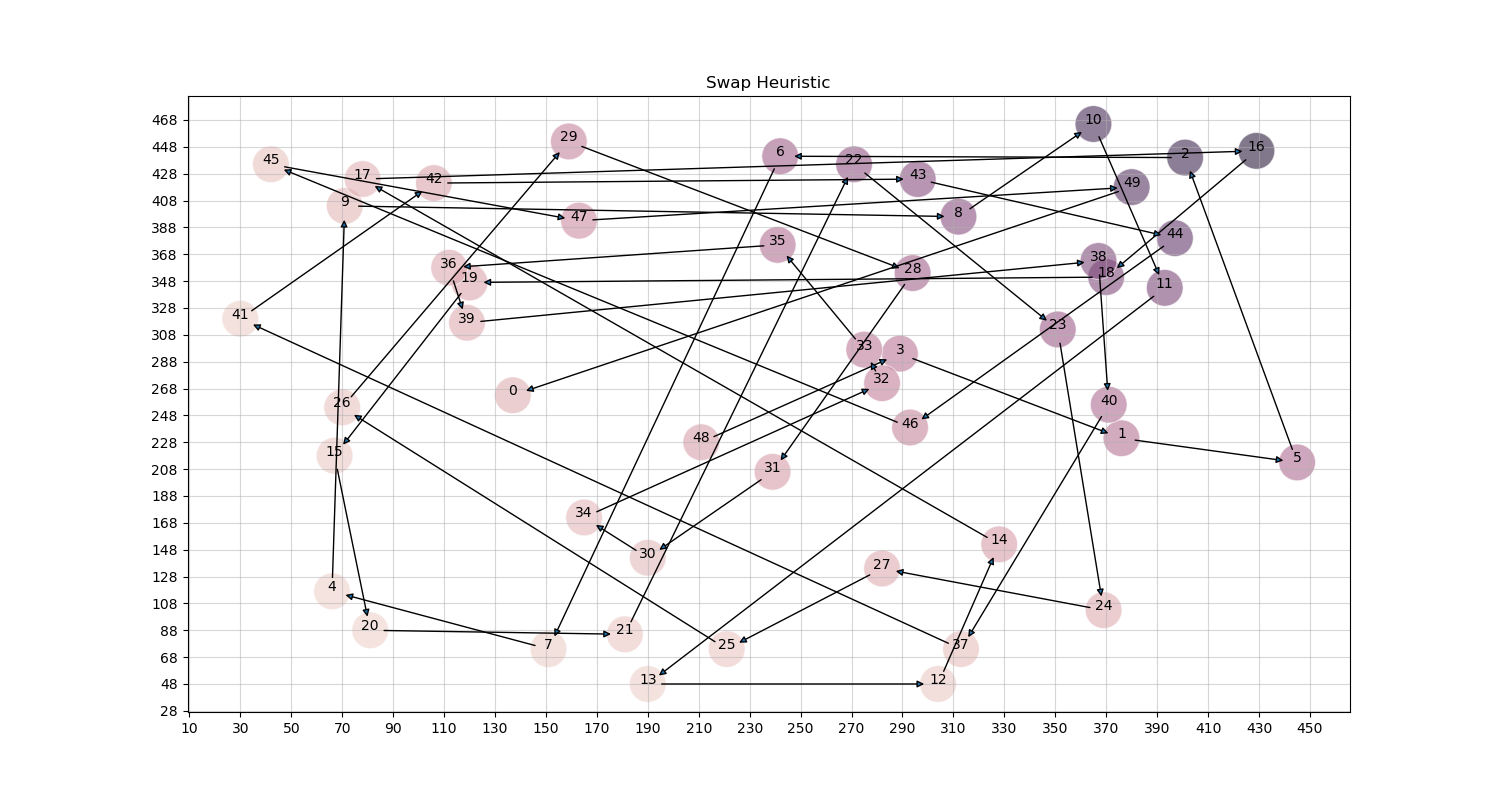
Travelling Salesman problem
Basic to elegant solutions for the travelling salesman problem. Includes Christofides 1.5 approximation algorithm. Also creates graphs and displays graphically for testing the algorithm.
-
Divy B
- December 17, 2023
Heuristics for the Travelling Salesman Problem
- Heuristics for the Travelling Salesman Problem
Introduction
The Traveling Salesman problem is quite interesting and is known to be NP-complete (Decision version). Solving it will lead to better results in a lot of its applications. I am not going to attempt it here to solve a general polynomial time algorithm, as that is beyond my skills now.
However, there are many versions of the problem, on which specifically we can design algorithms that might give better and faster results. Moreover, there are a lot of partial solutions available. Here, I will implement the most basic solutions leading up to the elegant ones.
Solutions
Swap Heuristic
Here, I explore the effect of repeatedly swapping the order in which a pair of adjacent cities are visited, as long as this swap improves (reduces) the cost of the overall tour. 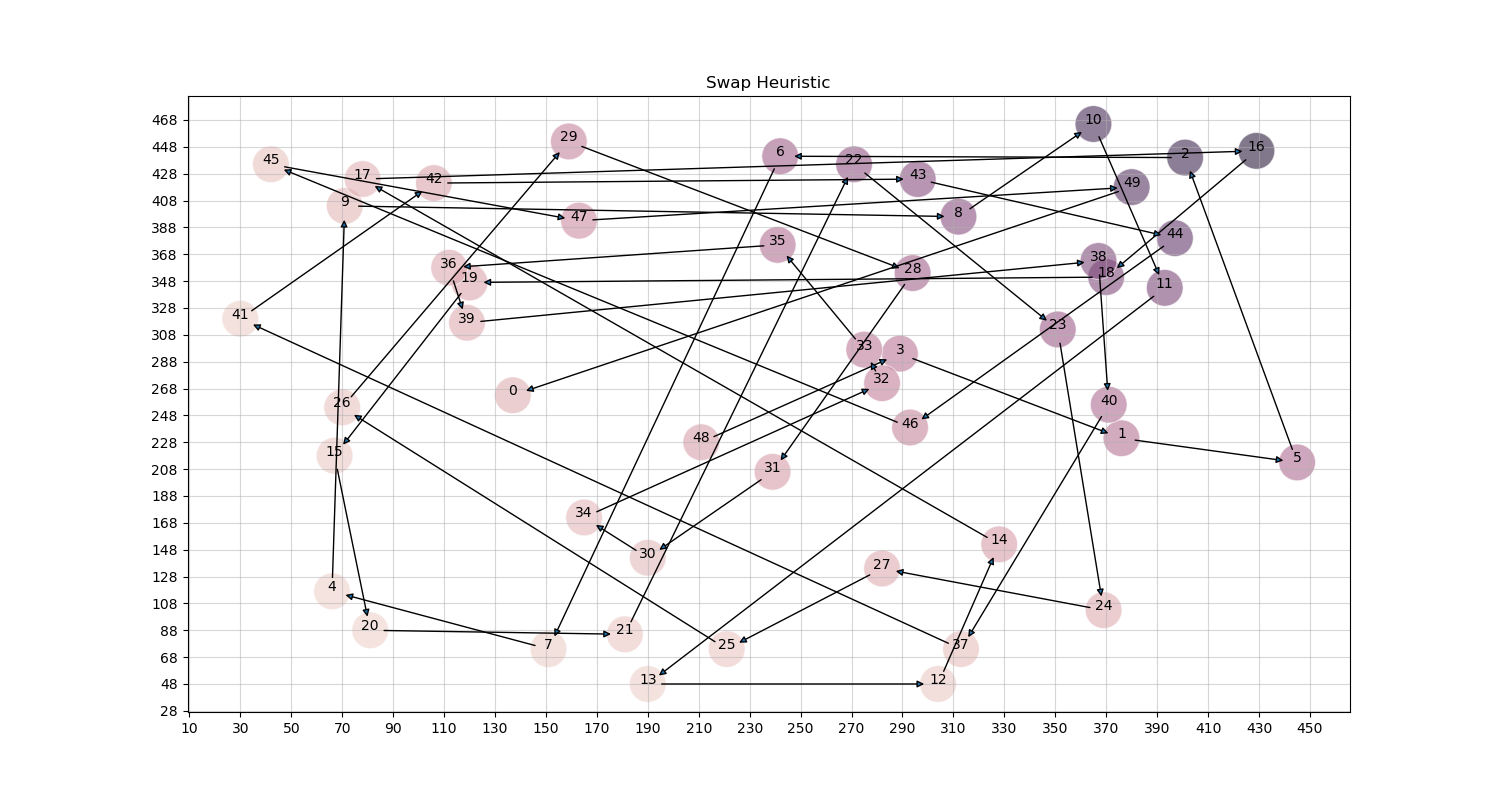
2-Opt Heuristic
The 2-Opt Heuristic is another heuristic that repeatedly makes local adjustments until there is no improvement from doing these. In this case, the alterations are more significant than the swaps.
This method repeatedly nominates a contiguous sequence of cities on the current tour and proposes that these be visited in the reverse order if that would reduce the overall cost of the tour.
Greedy
A commonly used approach to optimization problems is the greedy approach, where at each step we do what seems best, and hope that this will lead to a globally optimal solution.
For the TSP problem, this approach involves taking some initial city/node (for us, we will take the one indexed 0) and building a tour out from that starting point. At the i-th step (for i= 0,β¦), we consider the recently-assigned endpoint in the path against all previously unused nodes, and then we take our next node to be the one closest in distance to the node at i. This will eventually create
a permutation within the solution.
2-Approximation Algorithm
This is a bit sophisticated method, but requires the graph to follow the triangle inequality.
We start by computing an MST (minimum spanning tree) whose weight is a lower bound on the length of an optimal TSP tour. Then, use this MST to build a tour whose cost is no more than twice that of MSTβs weight as long as the cost function satisfies the triangle inequality.
This website provides a better explanation and mathematics proof for more details http://www.personal.kent.edu/~rmuhamma/Algorithms/MyAlgorithms/AproxAlgor/TSP/tsp.htm 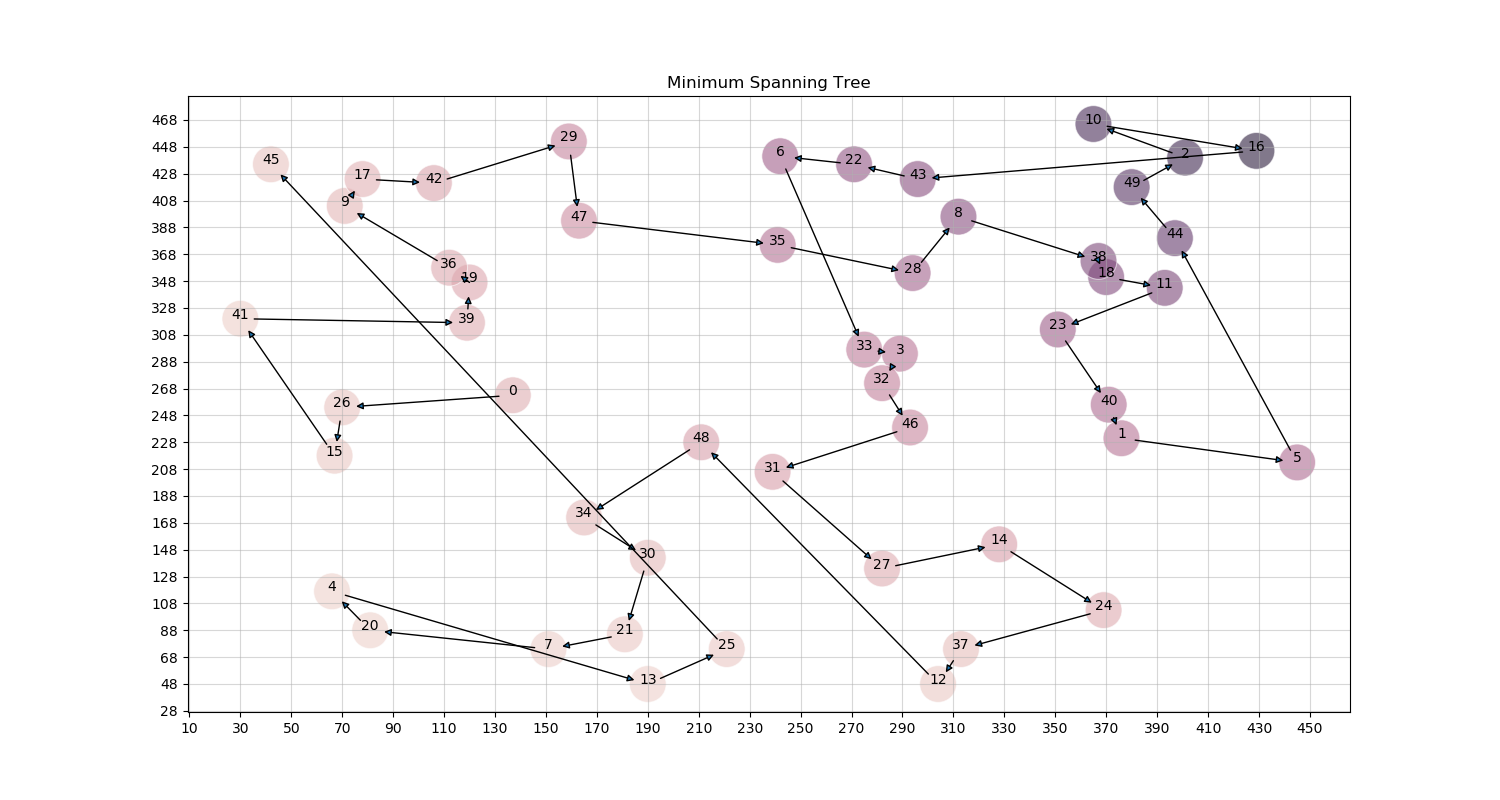
Christofides Algorithm
To tackle this, I did some research and found Christofideβs algorithm to be quite interesting which matched the above requirements. It claims to guarantee a solution within 1.5 errors.
Algorithm
It can be described in a few simple steps:
- Find the minimum spanning tree T of the graph.
- Find the Odd degree of vertices O. (They are even in number due to handshaking lemma.)
- Find the minimum weight perfectly matching M from the vertices O.
- Combine M and T to form an Eulerian circuit.
- Now form a Hamiltonian circuit from the circuit in the previous step.
Proof for polynomial time.
The following are the running times for each of the above steps.
- O(V2), I used Primβs algorithm designed for the adjacency matrix. Note, that it can be improved to O(E.log(V) if using heaps.
- O(V) Goes through all the vertices once.
- O(V2). Note, that this does not find the perfect minimum weight matching. I decided to implement a greedy solution which gives an approximation of the perfect minimum weight matching.
- O(V2). This just traverses the combined graph of M and T.
- O(V). This removes any extra edges that occur.
As we can see, the algorithm consists of combining steps 1-5, and so it runs in O(V2) time, which is polynomial. I have annotated the algorithm in the implementation properly for you to verify it.
Proof for 1.5 error approximation
The weight of a minimum spanning tree is less than the optimal solution:
- Take an optimal tour of cost OPT (optimal solution).
- Drop an edge to obtain a tree T.
- All distances are positive so cost(T) <= cost OPT, Hence cost(MST) <= cost OPT.
The two-approximation algorithm traverses the Minimum spanning tree twice, at most repeating all vertexes V, 2V times. Resulting in the cost to be doubled. Hence: $$ πππ π‘(πππ)<= πππ <=2.πππ π‘(πππ) $$ We improve this algorithm, by instead of traversing all the edge two times, we can make shortcuts between odd vertices. Therefore, the cost of the tour becomes: $$ πππ π‘(πππ)+πππ π‘(πππππππ‘ πππ‘πβπππ ππ) $$
$$ πππ π‘(πππ)+πππ π‘(ππ)β€πππ π‘(πππ)+πππ π‘(ππ) $$
Bounding, PM
- Take an optimal tour of cost OPT.
- Consider the odd vertices O.
- Shortcut the path to only use O.
- As they have perfect matchings, they partition into M1 and M2, such that cost(M1)+Cost(M2) <=OPT
- Therefore Cost(PM) <=OPT/2.
$$ πππ π‘(πππ)+πππ π‘(ππ)β€πππ π‘(πππ)+πππ π‘(πππ)/2 $$
Therefore, Christofideβs Algorithm is said to have a 1.5 approximation bound for TSP-metric problems.
Testing
I have written the details for testing, in the test.py file followed by the high-level description in the pdf files.
Here is the overview: